Quantum 101 - Eps 01: Qubits and Quantum Circuits
An introduction to quantum bits (qubits), quantum circuits and how to build a first simple quantum circuit using Qiskit.
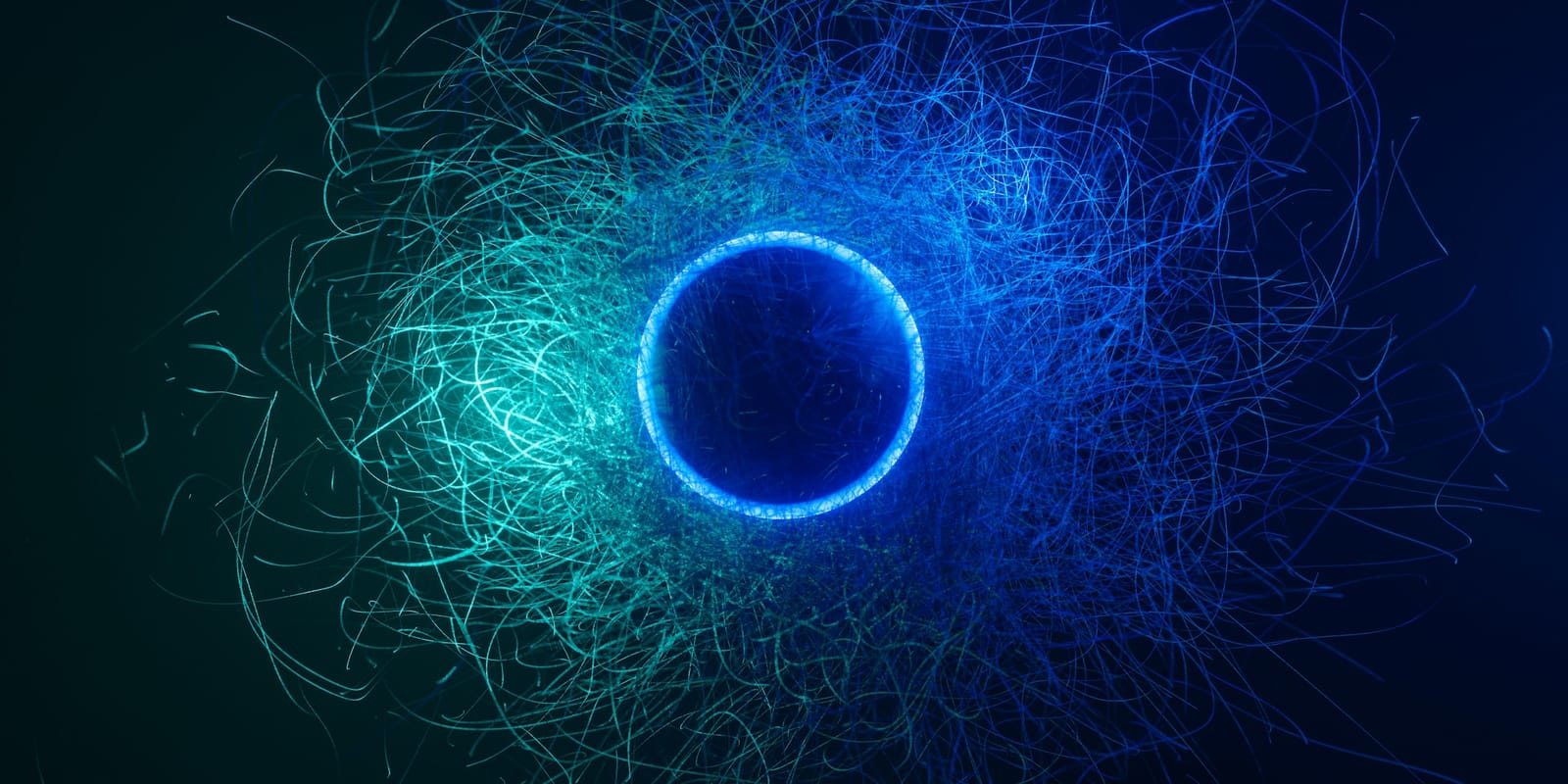
1. From Bits to Qubits
Bits and Classical Computers
A tool used for cutting or making holes?
A piece of metal you put in a horse's mouth?
The smallest unit of information?
→ Well, all right!
But, in computer science:
- A bit is the smallest (classical) unit of information that must be either 0 or 1.
- Bits can represent any piece of information. For example, we can represent the number \(2730_{10}\) in binary as \(101010101010_2\) , the character H as \(1001000_2\) , the symbol @ as \(1000000_2\) . More example here
- If you have \(n\) bits, they can be in \(2^n\) different classical states.
What is a (classical) computer?
- A computer is an electronic device for storing and processing data, typically in binary form (bits), according to instructions given to it in a variable program.
Qubits and Quantum Computers
What is a qubit?
- A qubit or quantum bit is the basic unit of quantum information, an extension of the bit to quantum mechanics.
- Qubit is a quantum variant of the bit, which means a qubit has exactly the same restrictions as a normal bit: it can store a single piece of information and can only give the output of 0 or 1. However, qubits can also be manipulated in ways that can only be described by quantum mechanics.
- It is a two-state quantum-mechanical system and one of the simplest systems displaying the peculiarity (essential characteristic) of quantum mechanics.
- A quantum state can be in superposition (i.e. simultaneously in 0 and 1). Superposition allows calculations to be performed on many states at the same time. So, some quantum algorithms have exponential speed-up.
What is a quantum computer?
Similar to a standard digital computer, which represents all information in the form of binary strings (or classical bits), the main difference between quantum computers and classical computers is that they use qubits instead of bits to represent information.
What is a quantum simulator?
A quantum simulator is a standard computer that calculates what an ideal quantum computer would do. Simulations are only possible for small numbers of qubits (~50 qubits). An example is the QASM Simulator by IBM Quantum.
2. Quantum Circuits at a glance
Computation as a Circuit
- Computation is the manipulation process to turn the inputs we have into the outputs we need (either using bits or qubits)
- A circuit diagram is a representation of the computation process.
Example: Two following bit-based and qubit-based circuit diagrams are equivalent
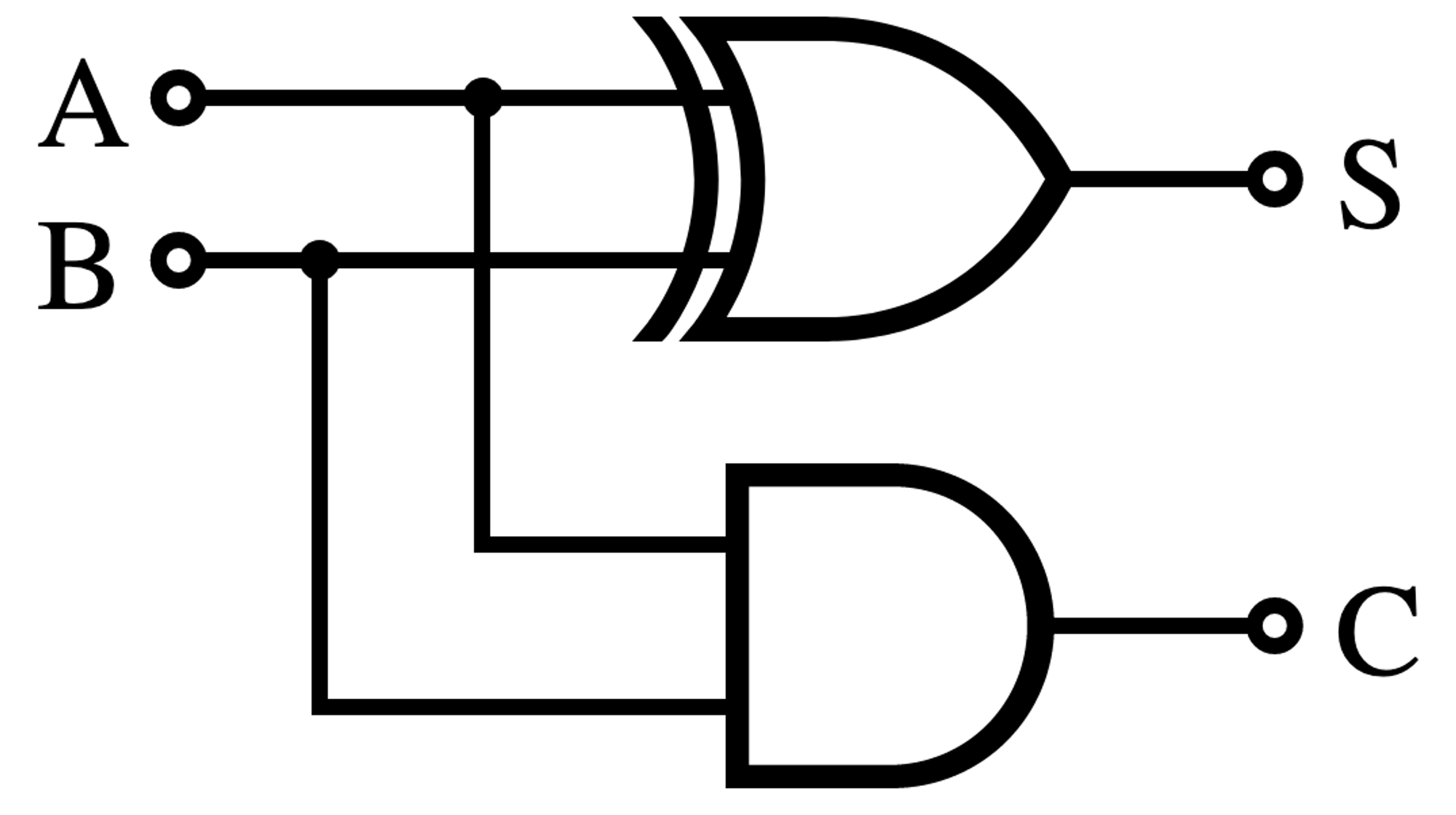
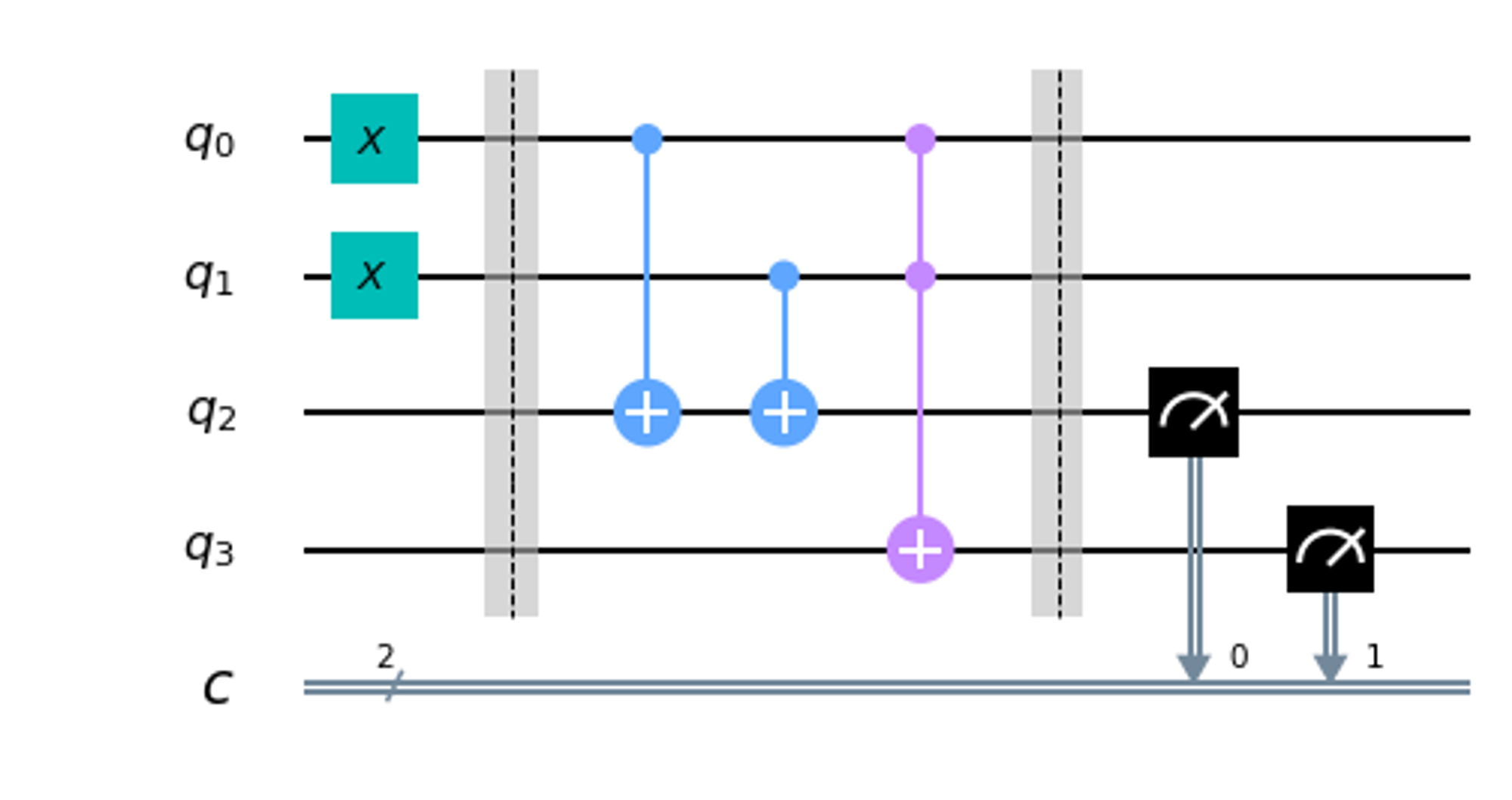
What is a Quantum circuit?
In a quantum circuit, we typically need to do 3 jobs:
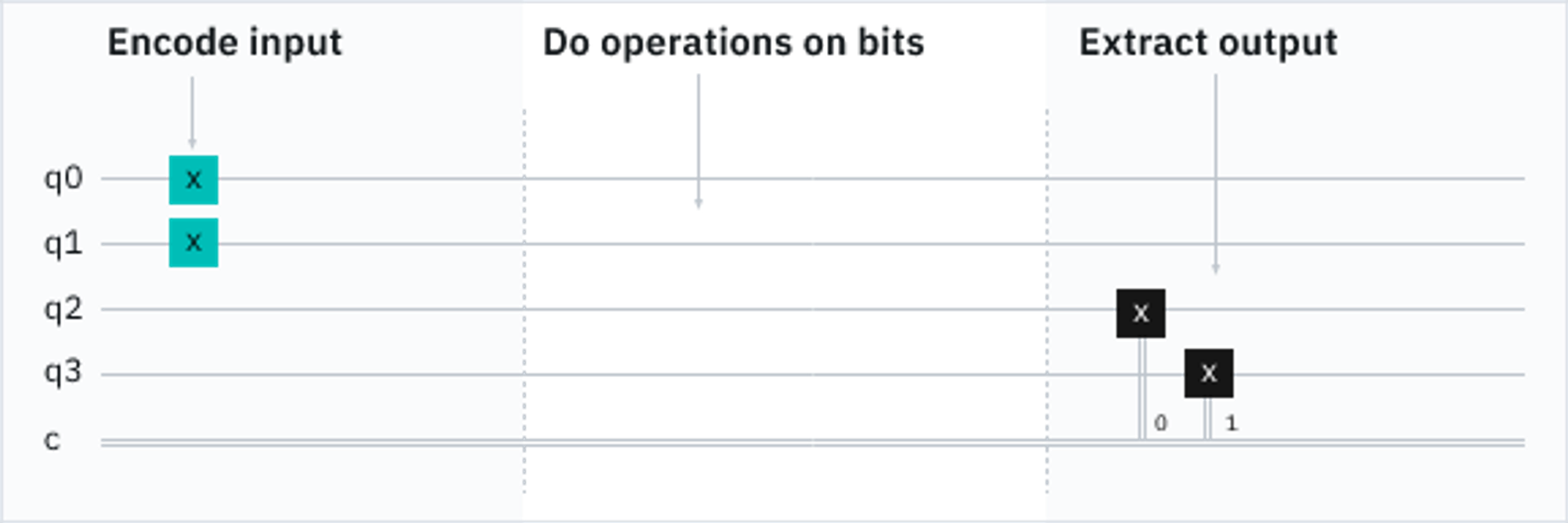
- Encode the Input
- Do some actual computation on the input
- Extract an output
Example: a First quantum circuit with Qiskit
n = 8
qc = QuantumCircuit(n)
qc.x(7)
for j in range(n):
qc.measure(j,j)
qc.draw()
- Encoding an input: Using NOT gate to flip final qubit (q7) from 0 to 1: \( 10000000 = q_7q_6q_5q_4q_3q_2q_1q_0\) (right to left)
Note: Qiskit numbers the bits from right to left. Why?
It has advantages when using bits to represent numbers. Qubit \(n^{th}\) is telling how many \(2^n\)s we have in the number. For example: \( 10000000 = 2^7 = 128 \) - Computation: Do nothing, keep the input unchanged
- Output: Extract the output by using measurement - 1000000
3. Example: Build our first Quantum Circuit to do the addition
What is the Half Adder?
The Half Adder is a type of combinational logic circuit that adds two of the 1-bit binary digits. It generates the carry and sum of both the inputs.
– The Half Adder does not add the carry obtained from the previous addition to the next one.– The logic circuits of Haft-Adder and Full-Adder– What is about the Full Adder?
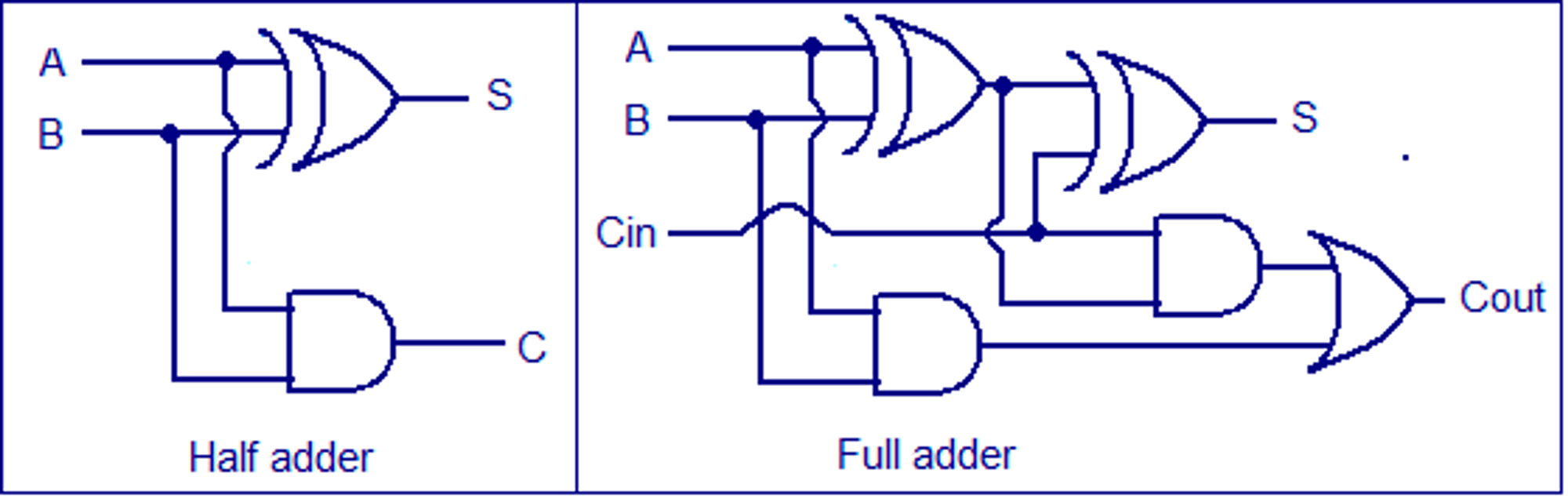
The Full Adder is also a type of combinational logic that adds three of the 1-bit binary digits for performing an addition operation. It generates a sum of all three inputs along with a carrying value.– The Full Adder, along with its current inputs A and B, also adds the previous carry.
Key ideas of the Half Adder
- Four basic sums:
0+0 = 00 (in decimal, this is 0+0=0)
0+1 = 01 (in decimal, this is 0+1=1)
1+0 = 01 (in decimal, this is 1+0=1)
1+1 = 10 (in decimal, this is 1+1=2) - Key ideas of Half Adder:
– The rightmost bit is completely determined by whether the two bits we are adding are the same or different.Specifically, if the two bits are equal (i.e., 0 + 0 or 1+1), the rightmost bit of the answer comes out 0 and otherwise.
→ Solution: Use the XOR gate (or CNOT gate in quantum computation)
– The leftmost bit is only 1 if both of the bits we are adding are 1
→ Solution: Using the AND gate (or Toffoli gate in quantum computation)– We don’t want to overwrite the input
→ Solution: Using a different pair of qubits to write the output
What are an XOR and a CNOT gate?
- An XOR gate implements an exclusive or; that is, a 1 output results if one, and only one, of the inputs to the gate is 1. If both inputs are 1 or both are 0, the output result is 0.
A CNOT (Controlled-NOT) gate is a (reversible) quantum version of an XOR gate
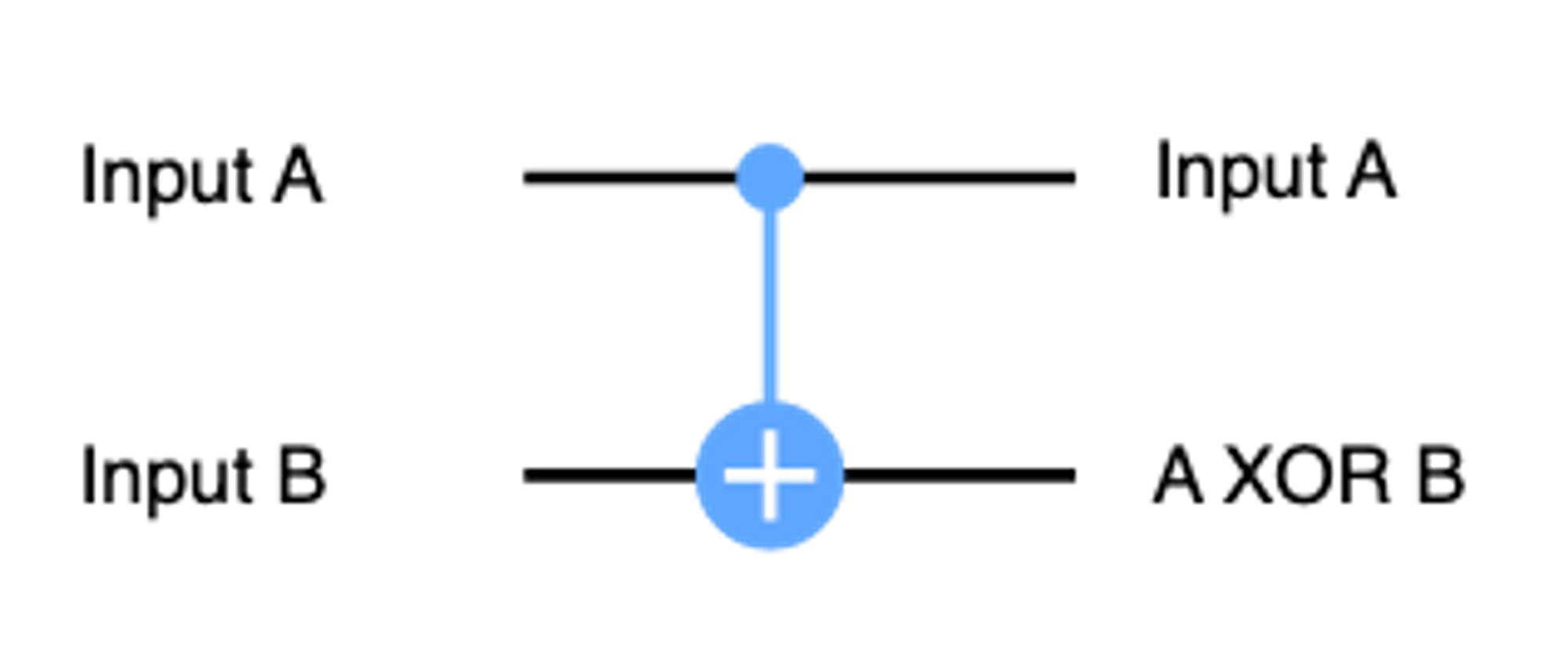
– CNOT gate applies to a pair of qubits. One acts as the Control qubit (with the little dot, Input A), and another acts as the target qubit (with the big circle and +, Input B)– If the control qubit is 1, the CNOT gate does a NOT (flip) on the target qubit. Otherwise, it does nothing.
What are an AND and a Tofolli gate?
- An AND gate implements logical conjunction (∧) from mathematical logic. If (and only if) both inputs are 1, the output result is 1; otherwise, the output is 0
- The Toffoli gate, invented by Tommaso Toffoli, is a universal reversible logic gate, which means that any reversible circuit can be constructed from Toffoli gates. It is also known as the controlled-controlled-not gate, which describes its action. It has 3-bit inputs and outputs; if the first two bits are both set to 1, it inverts the third bit, otherwise, all bits stay the same.
- The Toffoli gate is essentially the atom of mathematics. It is the simplest element, from which every other problem-solving technique can be compiled.
Implement the Quantum Half Adder circuit
Example: The input is 11 (perform the 1 + 1 addition)
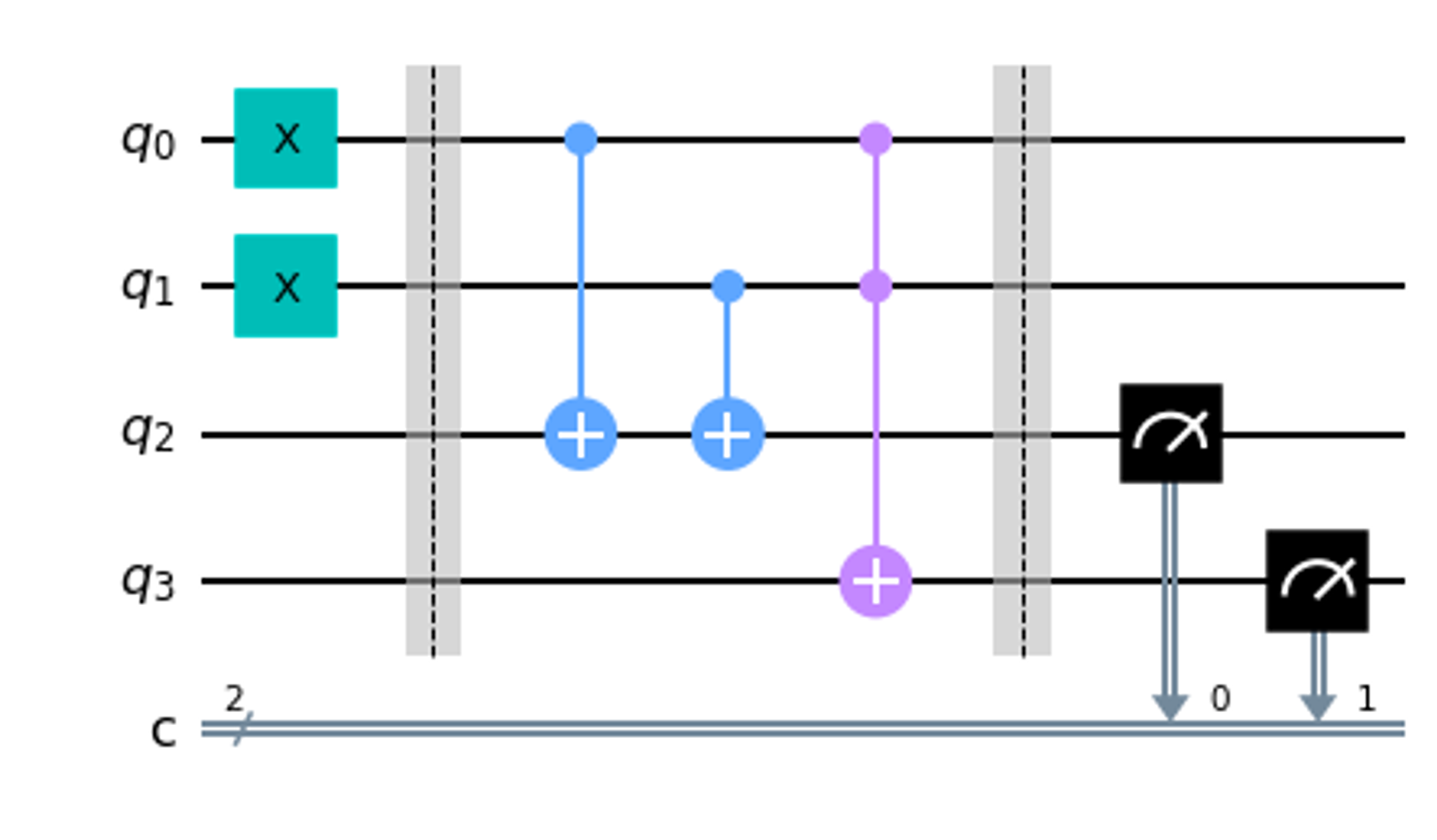
Qiskit code:
qc_ha = QuantumCircuit(4,2)
# encode inputs in qubits 0 and 1
qc_ha.x(0) # For a=0, remove the this line. For a=1, leave it.
qc_ha.x(1) # For b=0, remove the this line. For b=1, leave it.
qc_ha.barrier()
# use cnots to write the XOR of the inputs on qubit 2
qc_ha.cx(0,2)
qc_ha.cx(1,2)
# use ccx to write the AND of the inputs on qubit 3
qc_ha.ccx(0,1,3)
qc_ha.barrier()
# extract outputs
qc_ha.measure(2,0) # extract XOR value
qc_ha.measure(3,1) # extract AND value
qc_ha.draw()
Simulate and get the result (Qiskit <1.x):
counts = execute(qc_ha,Aer.get_backend('qasm_simulator')).result().get_counts()
plot_histogram(counts)
Result: 1 + 1 = 10 (binary). Or 1 + 1 = 2 (decimal)
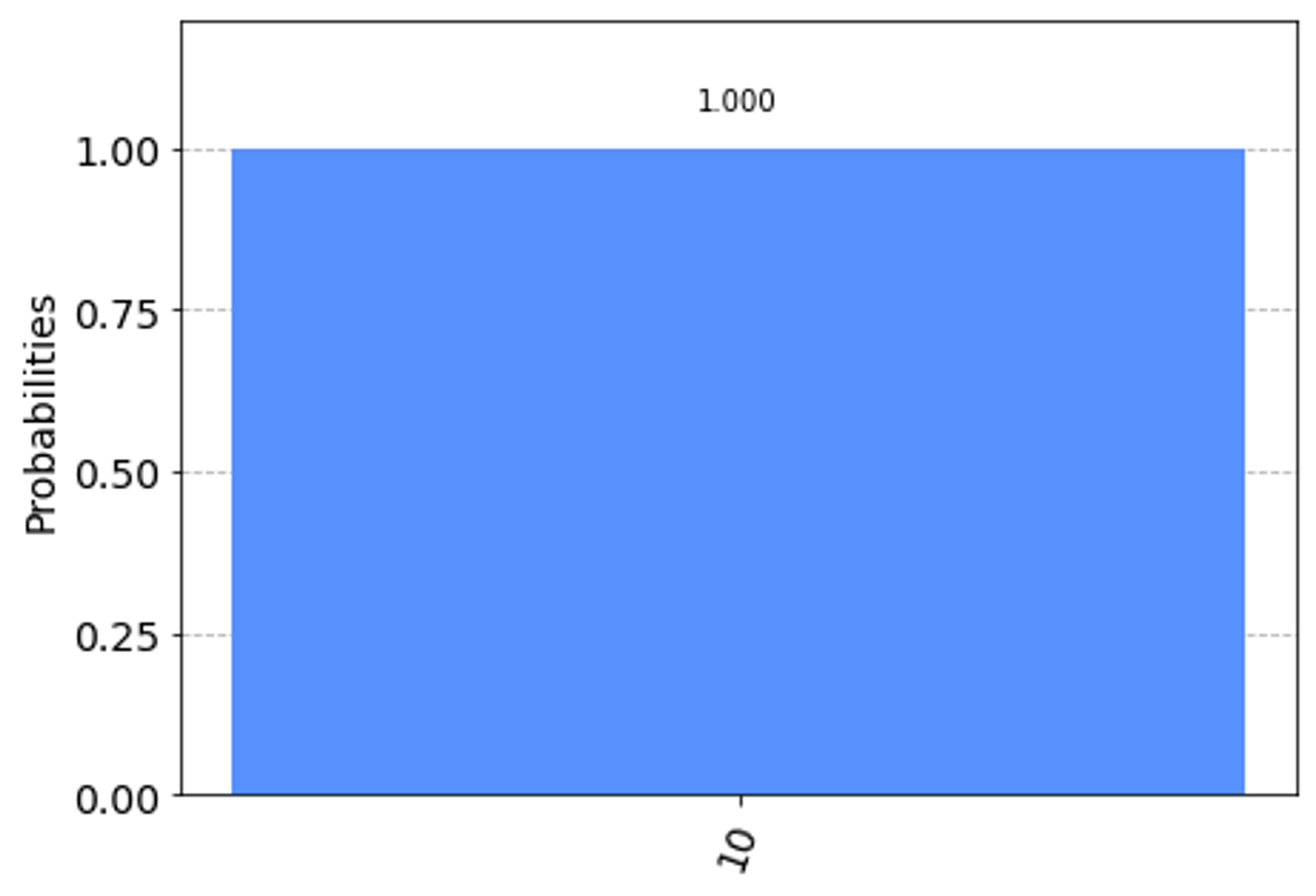
References
1 Qiskit Online Textbook - Quantum States and Qubits by IBM Quantum (https://learn.qiskit.org/course/ch-states)
2 IBM Quantum Summer School 2021 (https://learn.qiskit.org/summer-school/2021)
2 Quantum Half-adder and Full-adder by Lahiru Madushanka (https://lahirumadushankablog.wordpress.com/2020/02/04/quantum-half-adder-and-full-adder/)